83
|
1 # garden.contextmenu |
|
2 |
|
3 Collection of classes for easy creating **context** and **application** menus. |
|
4 |
|
5 ## Context Menu |
|
6 |
|
7 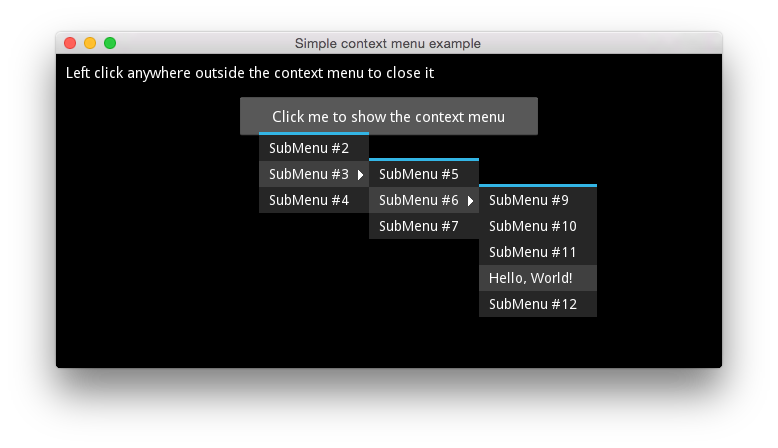 |
|
8 |
|
9 Context menu is represented by `ContextMenu` widget that wraps all menu items as `ContextMenuTextItem` widgets. Context menus can be nested, each `ContextMenuTextItem` can contain maximum one `ContextMenu` widget. |
|
10 |
|
11 ```python |
|
12 import kivy |
|
13 from kivy.app import App |
|
14 from kivy.lang import Builder |
|
15 import kivy.garden.contextmenu |
|
16 |
|
17 kv = """ |
|
18 FloatLayout: |
|
19 id: layout |
|
20 Label: |
|
21 pos: 10, self.parent.height - self.height - 10 |
|
22 text: "Left click anywhere outside the context menu to close it" |
|
23 size_hint: None, None |
|
24 size: self.texture_size |
|
25 |
|
26 Button: |
|
27 size_hint: None, None |
|
28 pos_hint: {"center_x": 0.5, "center_y": 0.8 } |
|
29 size: 300, 40 |
|
30 text: "Click me to show the context menu" |
|
31 on_release: context_menu.show(*app.root_window.mouse_pos) |
|
32 |
|
33 ContextMenu: |
|
34 id: context_menu |
|
35 visible: False |
|
36 cancel_handler_widget: layout |
|
37 |
|
38 ContextMenuTextItem: |
|
39 text: "SubMenu #2" |
|
40 ContextMenuTextItem: |
|
41 text: "SubMenu #3" |
|
42 ContextMenu: |
|
43 ContextMenuTextItem: |
|
44 text: "SubMenu #5" |
|
45 ContextMenuTextItem: |
|
46 text: "SubMenu #6" |
|
47 ContextMenu: |
|
48 ContextMenuTextItem: |
|
49 text: "SubMenu #9" |
|
50 ContextMenuTextItem: |
|
51 text: "SubMenu #10" |
|
52 ContextMenuTextItem: |
|
53 text: "SubMenu #11" |
|
54 ContextMenuTextItem: |
|
55 text: "Hello, World!" |
|
56 on_release: app.say_hello(self.text) |
|
57 ContextMenuTextItem: |
|
58 text: "SubMenu #12" |
|
59 ContextMenuTextItem: |
|
60 text: "SubMenu #7" |
|
61 ContextMenuTextItem: |
|
62 text: "SubMenu #4" |
|
63 """ |
|
64 |
|
65 class MyApp(App): |
|
66 def build(self): |
|
67 self.title = 'Simple context menu example' |
|
68 return Builder.load_string(kv) |
|
69 |
|
70 def say_hello(self, text): |
|
71 print(text) |
|
72 self.root.ids['context_menu'].hide() |
|
73 |
|
74 if __name__ == '__main__': |
|
75 MyApp().run() |
|
76 ``` |
|
77 |
|
78 Arrows that symbolize that an item has sub menu is created automatically. `ContextMenuTextItem` inherits from [ButtonBehavior](http://kivy.org/docs/api-kivy.uix.behaviors.html#kivy.uix.behaviors.ButtonBehavior) so you can use `on_release` to bind actions to it. |
|
79 |
|
80 The root context menu can use `cancel_handler_widget` parameter. This adds `on_touch_down` event to it that closes the menu when you click anywhere outside the menu. |
|
81 |
|
82 |
|
83 ## Application Menu |
|
84 |
|
85 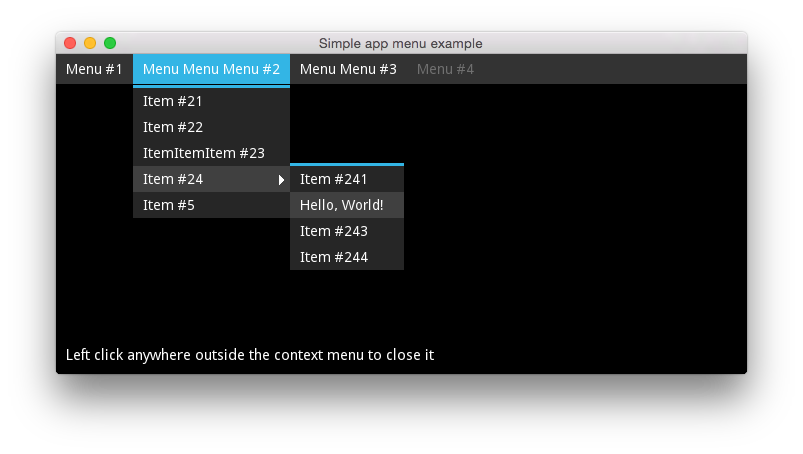 |
|
86 |
|
87 Creating application menus is very similar to context menus. Use `AppMenu` and `AppMenuTextItem` widgets to create the top level menu. Then each `AppMenuTextItem` can contain one `ContextMenu` widget as we saw above. `AppMenuTextItem` without `ContextMenu` are disabled by default |
|
88 |
|
89 ```python |
|
90 import kivy |
|
91 from kivy.app import App |
|
92 from kivy.lang import Builder |
|
93 import kivy.garden.contextmenu |
|
94 |
|
95 kv = """ |
|
96 FloatLayout: |
|
97 id: layout |
|
98 AppMenu: |
|
99 id: app_menu |
|
100 top: root.height |
|
101 cancel_handler_widget: layout |
|
102 |
|
103 AppMenuTextItem: |
|
104 text: "Menu #1" |
|
105 ContextMenu: |
|
106 ContextMenuTextItem: |
|
107 text: "Item #11" |
|
108 ContextMenuTextItem: |
|
109 text: "Item #12" |
|
110 AppMenuTextItem: |
|
111 text: "Menu Menu Menu #2" |
|
112 ContextMenu: |
|
113 ContextMenuTextItem: |
|
114 text: "Item #21" |
|
115 ContextMenuTextItem: |
|
116 text: "Item #22" |
|
117 ContextMenuTextItem: |
|
118 text: "ItemItemItem #23" |
|
119 ContextMenuTextItem: |
|
120 text: "Item #24" |
|
121 ContextMenu: |
|
122 ContextMenuTextItem: |
|
123 text: "Item #241" |
|
124 ContextMenuTextItem: |
|
125 text: "Hello, World!" |
|
126 on_release: app.say_hello(self.text) |
|
127 # ... |
|
128 ContextMenuTextItem: |
|
129 text: "Item #5" |
|
130 AppMenuTextItem: |
|
131 text: "Menu Menu #3" |
|
132 ContextMenu: |
|
133 ContextMenuTextItem: |
|
134 text: "SubMenu #31" |
|
135 ContextMenuDivider: |
|
136 ContextMenuTextItem: |
|
137 text: "SubMenu #32" |
|
138 # ... |
|
139 AppMenuTextItem: |
|
140 text: "Menu #4" |
|
141 # ... |
|
142 # The rest follows as usually |
|
143 """ |
|
144 |
|
145 class MyApp(App): |
|
146 def build(self): |
|
147 self.title = 'Simple app menu example' |
|
148 return Builder.load_string(kv) |
|
149 |
|
150 def say_hello(self, text): |
|
151 print(text) |
|
152 self.root.ids['app_menu'].close_all() |
|
153 |
|
154 if __name__ == '__main__': |
|
155 MyApp().run() |
|
156 ``` |
|
157 |
|
158 ## All classes |
|
159 |
|
160 `garden.contextmenu` provides you with a set of classes and mixins for creating your own customised menu items for both context and application menus. |
|
161 |
|
162 ### context_menu.AbstractMenu |
|
163 |
|
164 Mixin class that represents basic functionality for all menus. It cannot be used by itself and needs to be extended with a layout. Provides `cancel_handler_widget` property. See [AppMenu](https://github.com/kivy-garden/garden.contextmenu/blob/master/app_menu.py) or [ContextMenu](https://github.com/kivy-garden/garden.contextmenu/blob/master/context_menu.py). |
|
165 |
|
166 ### context_menu.ContextMenu |
|
167 |
|
168 Implementation of a context menu. |
|
169 |
|
170 ### context_menu.AbstractMenuItem |
|
171 |
|
172 Mixin class that represents a single menu item. Needs to be extended to be any useful. It's a base class for all menu items for both context and application menus. |
|
173 |
|
174 If you want to extend this class you need to override the `content_width` property which tells the parent `ContextMenu` what is the expected width of this item. It needs to know this to set it's own width. |
|
175 |
|
176 ### context_menu.ContextMenuItem |
|
177 |
|
178 Single context menu item. Automatically draws an arrow if contains a `ContextMenu` children. If you want to create a custom menu item extend this class. |
|
179 |
|
180 ### context_menu.AbstractMenuItemHoverable |
|
181 |
|
182 Mixin class that makes any class that inherits `ContextMenuItem` to change background color on mouse hover. |
|
183 |
|
184 ### context_menu.ContextMenuText |
|
185 |
|
186 Menu item with `Label` widget without any extra functionality. |
|
187 |
|
188 ### context_menu.ContextMenuDivider |
|
189 |
|
190 Menu widget that splits two parts of a context/app menu. |
|
191 |
|
192 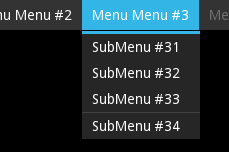 |
|
193 |
|
194 It also contains an instance of `Label` which is not visible if you don't set it any text. |
|
195 |
|
196 ```python |
|
197 ContextMenuTextItem: |
|
198 text: "SubMenu #33" |
|
199 ContextMenuDivider: |
|
200 text: "More options" |
|
201 ContextMenuTextItem: |
|
202 text: "SubMenu #34" |
|
203 ``` |
|
204 |
|
205 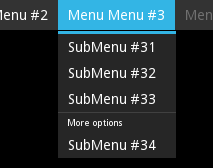 |
|
206 |
|
207 ### context_menu.ContextMenuTextItem |
|
208 |
|
209 Menu item with text. You'll be most of the time just fine using this class for all your menu items. You can also see it used in [all examples here](https://github.com/kivy-garden/garden.contextmenu/tree/master/examples). Contains a `Label` widget and copies `text`, `font_size` and `color` properties to it automatically. |
|
210 |
|
211 ### app_menu.AppMenu |
|
212 |
|
213 Application menu widget. By default it fills the entire parent's width. |
|
214 |
|
215 ### app_menu.AppMenuTextItem |
|
216 |
|
217 Application menu item width text. Contains a `Label` widget and copies `text`, `font_size` and `color` properties to it automatically. |
|
218 |
|
219 # License |
|
220 |
|
221 garden.contextmenu is licensed under MIT license. |